Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 교착 상태
- Multi-level Queue
- 유저 모드의 동기화
- The DIning Philosopher Problem
- Activity
- 모니터(Monitor)
- Stack영역
- 커널 모드
- 문맥 교환
- Light Weight Process
- 방금 그 곡
- The Banker's Algorithm
- 은행원 알고리즘
- 스레드 동기화
- Non-Preemptive
- 프로세스 제어 블록
- 임계 구역
- Heap영역
- Process Control Block
- 경량 프로세스
- Reentrant
- 동기 비동기
- 뮤텍스(Mutex)
- 커널 모드의 동기화
- 인터락 함수
- 프로세스
- 블로킹 논블로킹
- 프로세스 상태 전이도
- 유저 모드
- 스레드
Archives
Blog For Me
[자료구조와 알고리즘] 그래프 순회 - 너비 우선 탐색 (bfs) 본문
bfs 탐색
- 시작 정점 v를 방문한다.
- v에 인접한 모든 정점들을 방문한다.
- 새롭게 방문한 정점들에 인접하면서 아직 방문하지 못한 정점들을 방문한다.
- 인접한 정점들에 대해서 차례로 다시 너비 우선 탐색을 반복해야 하므로 선입선출의 구조를 갖는 Queue를 사용한다.
bfs 탐색 예시
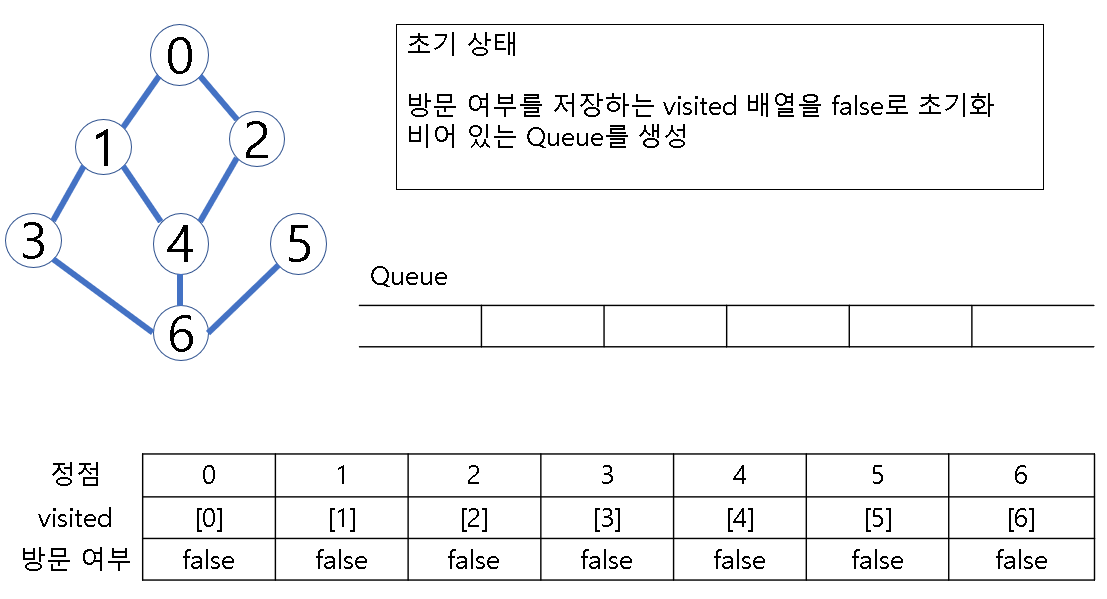
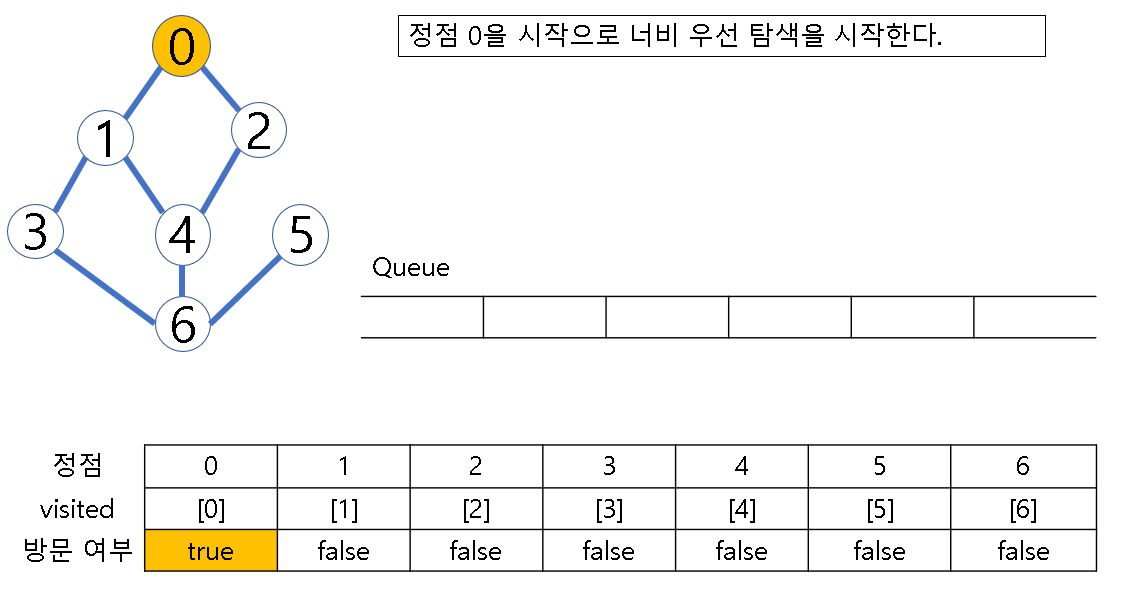
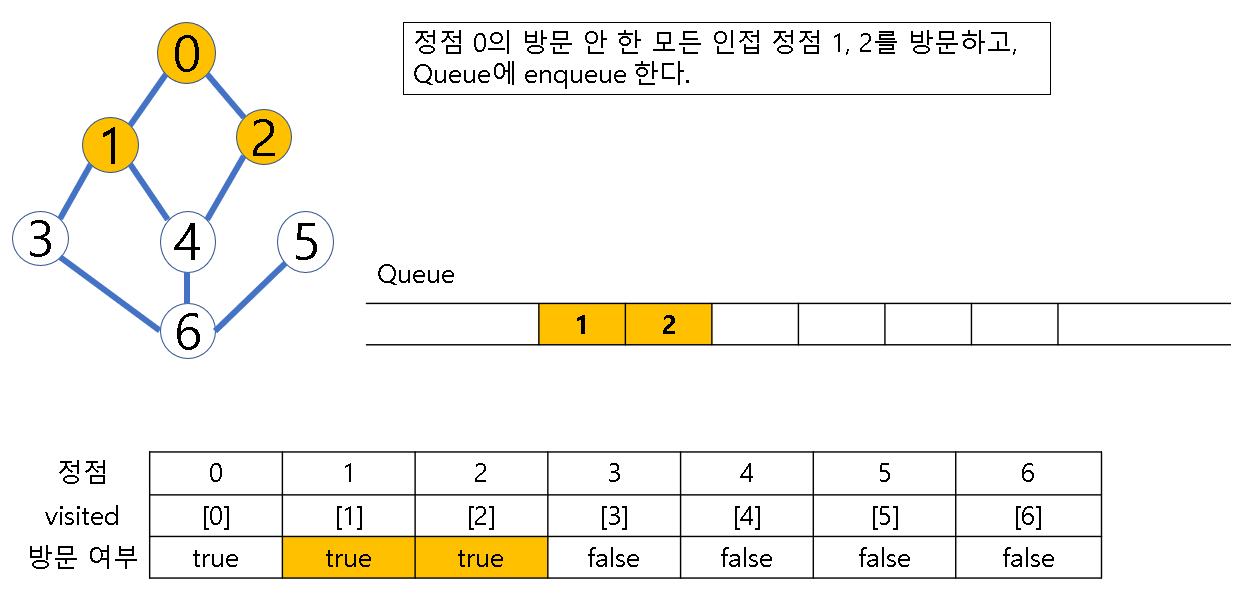
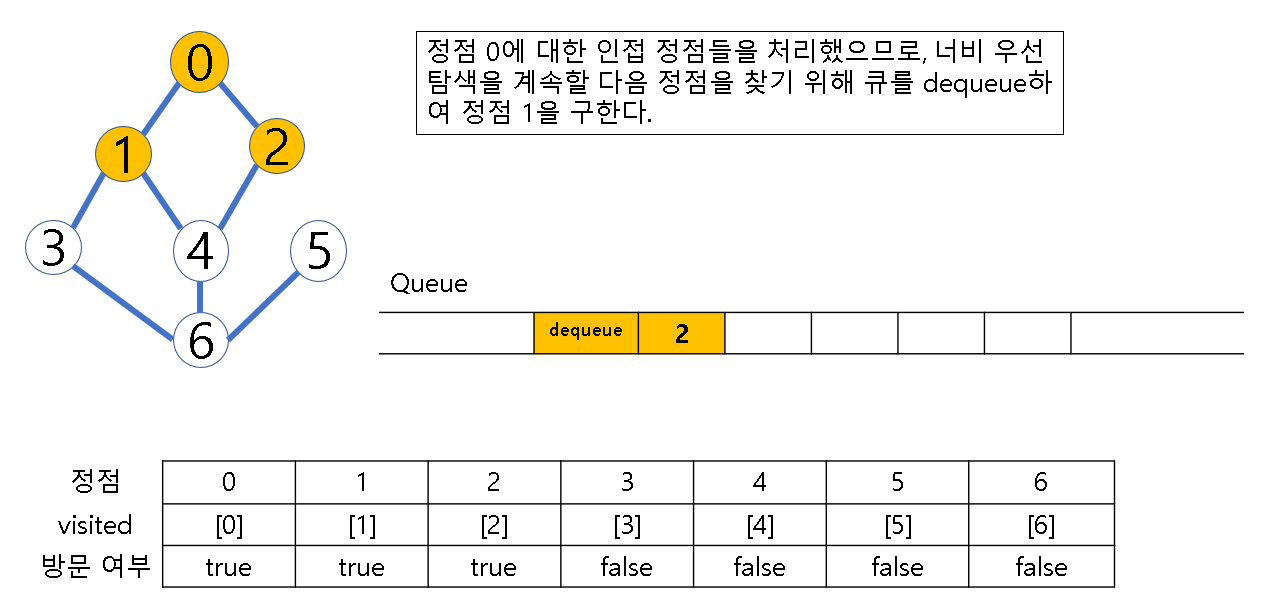
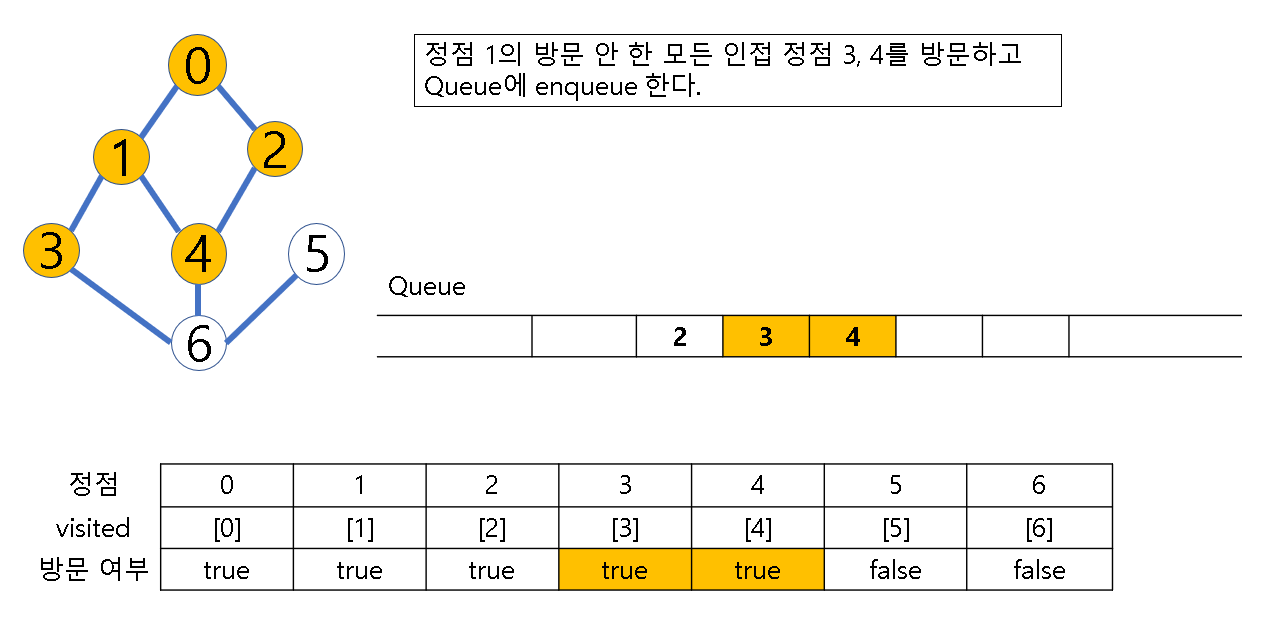
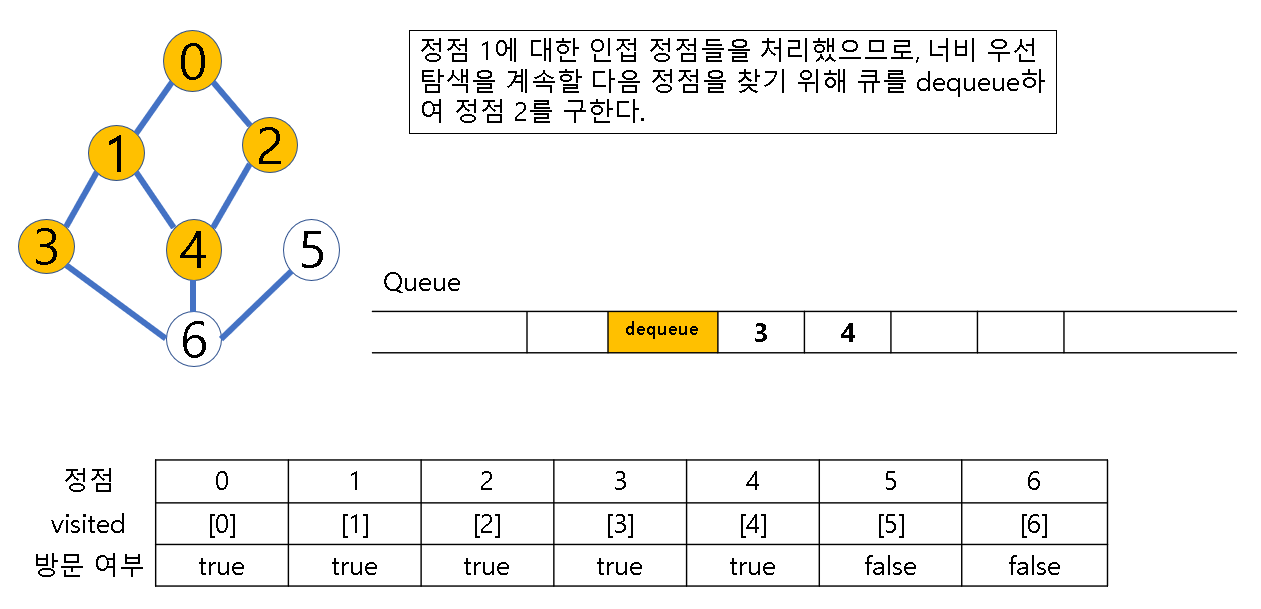
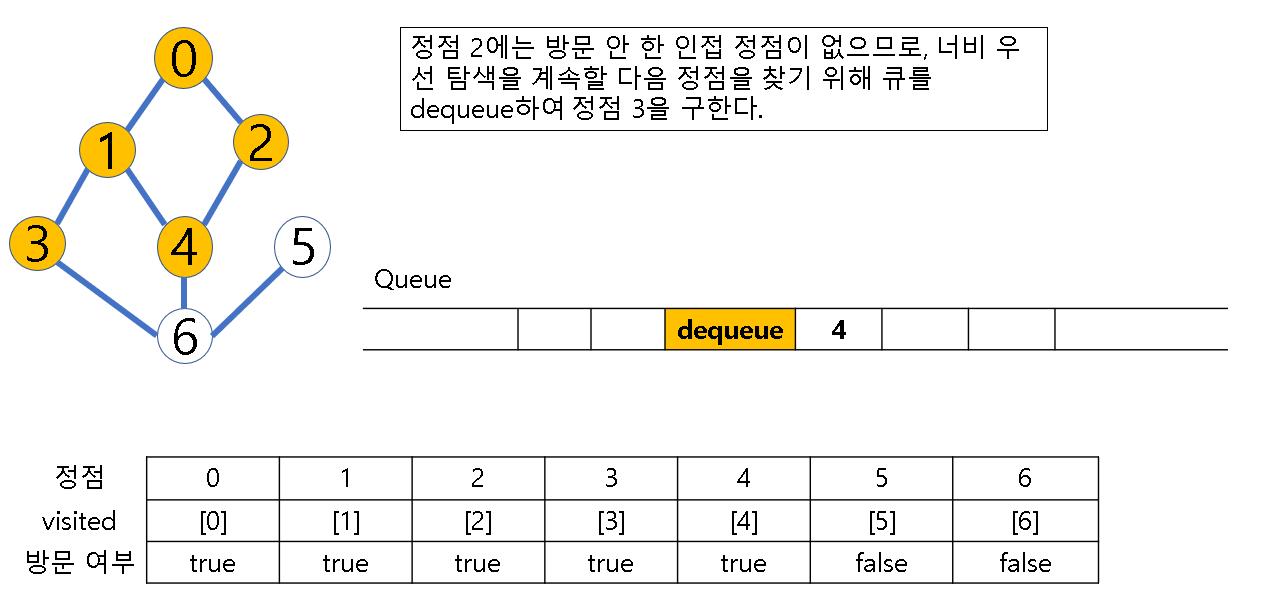
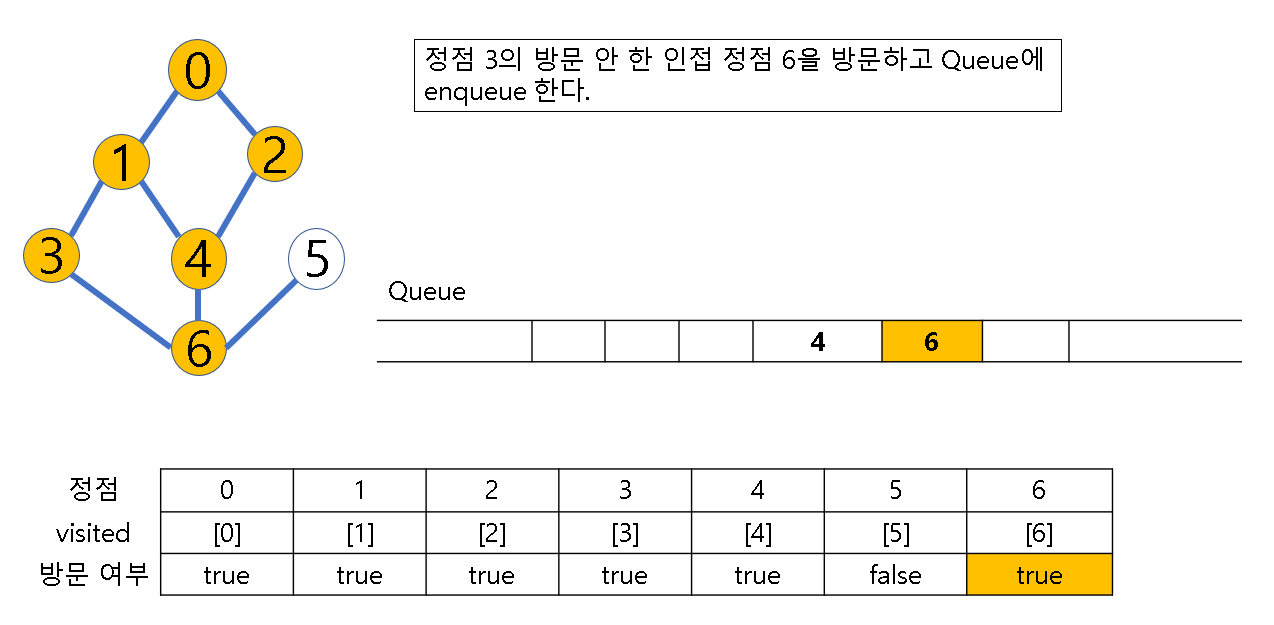
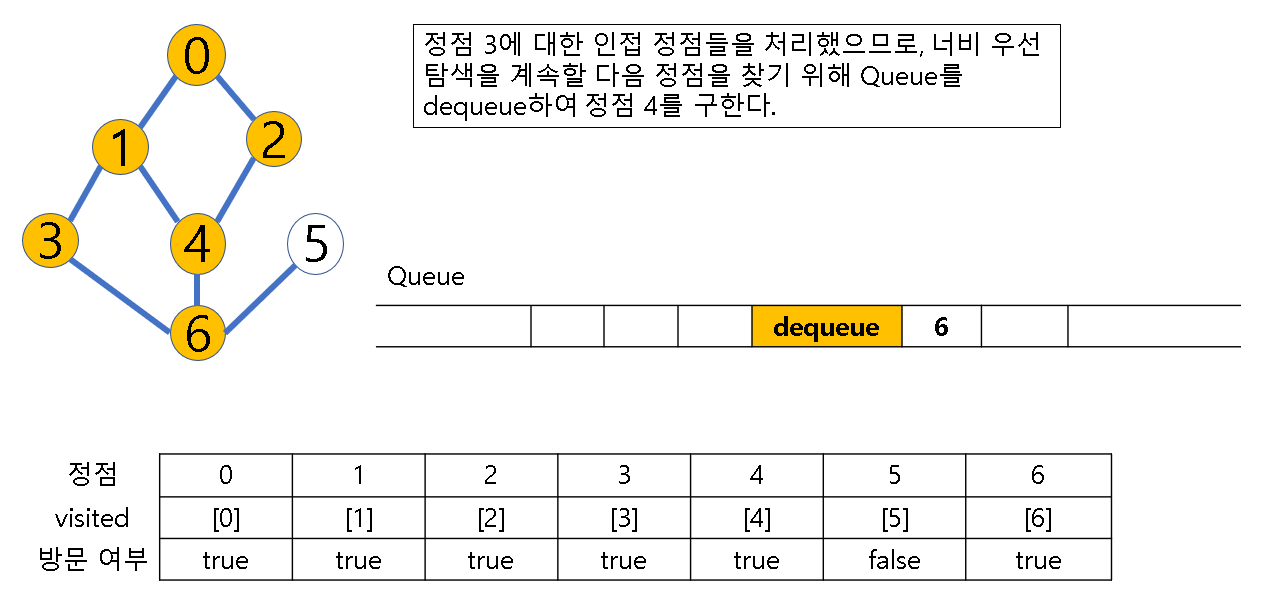
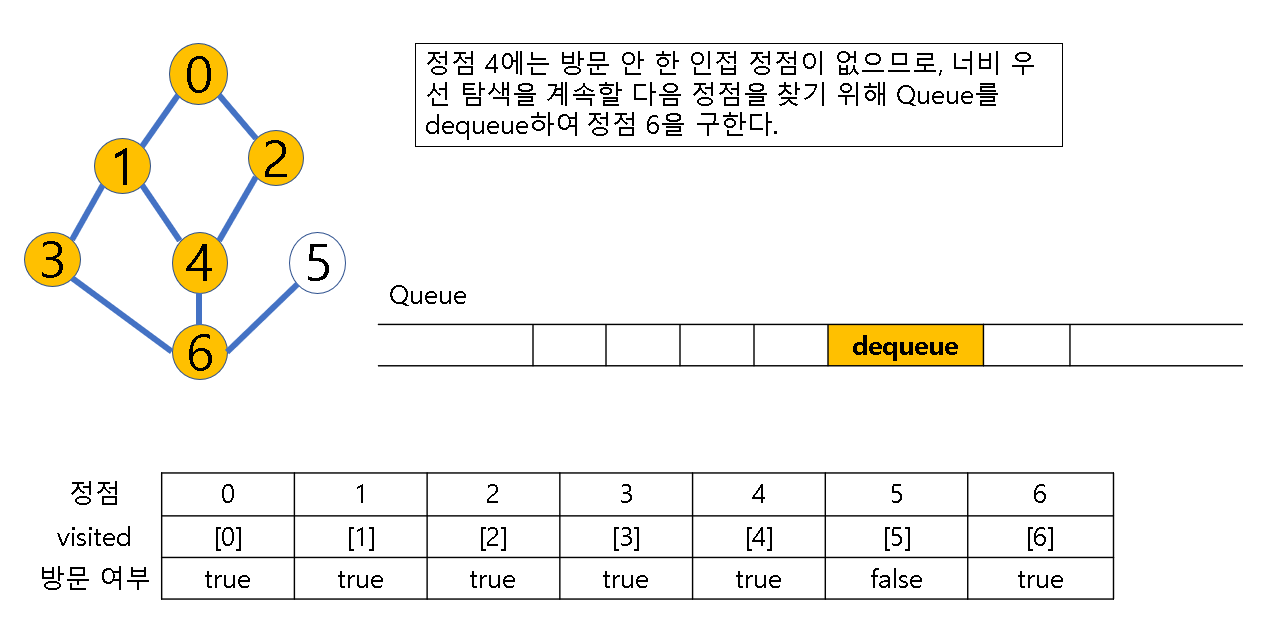
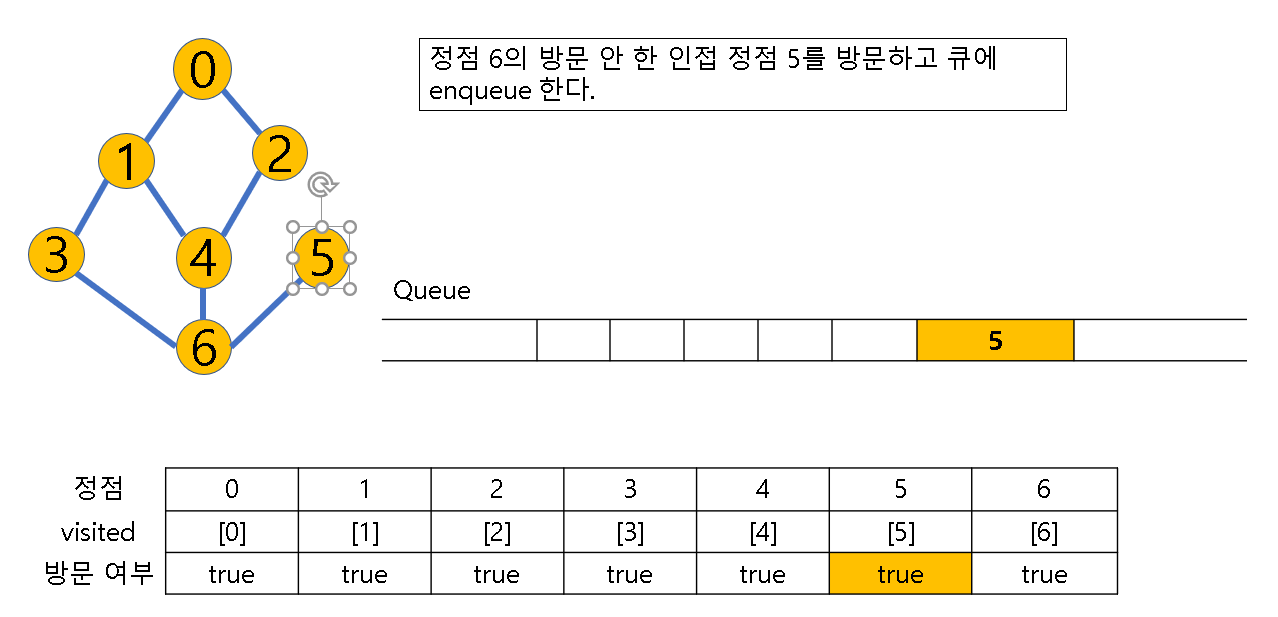
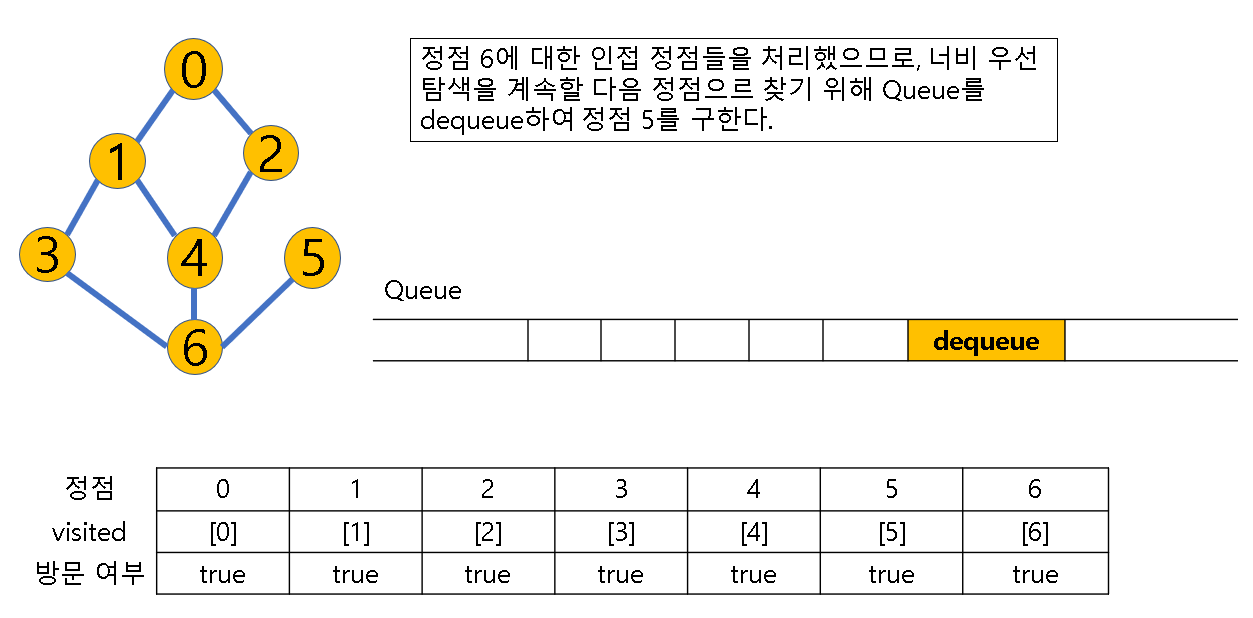
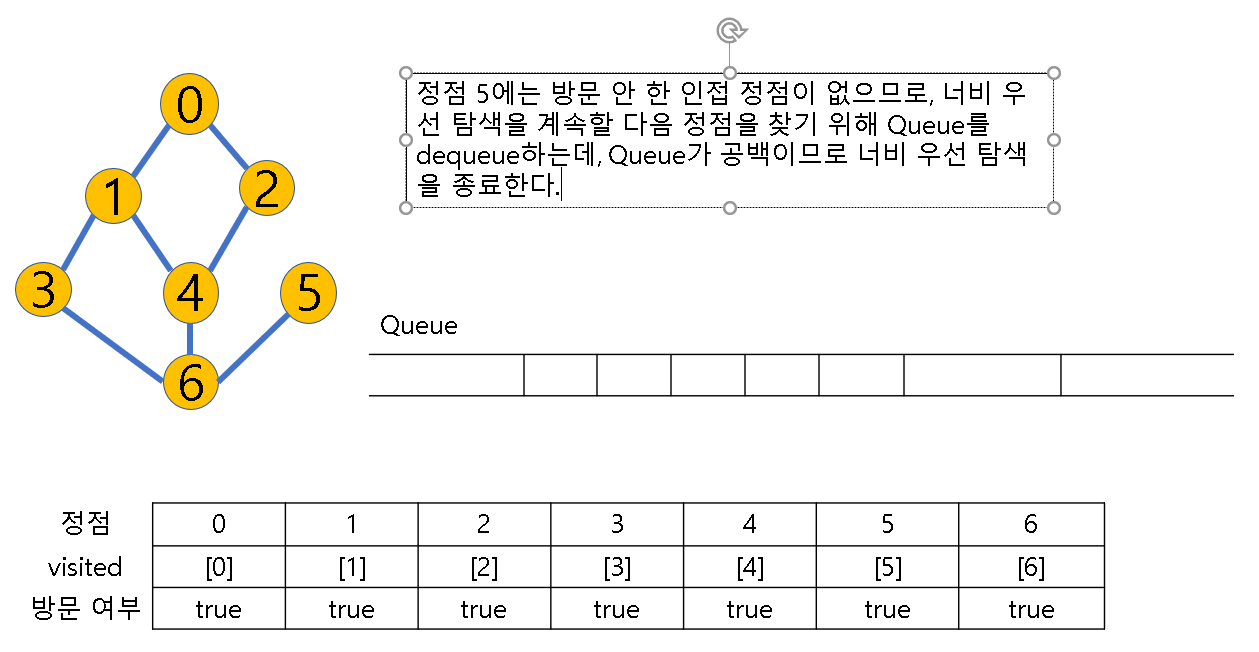
bfs 소스코드
class Graph{
private ArrayList<ArrayList<Integer>> edges;
private boolean[] visited;
public Graph(int size) {
this.edges = new ArrayList<ArrayList<Integer>>();
this.visited = new boolean[size];
for(int i=0;i<=size;i++) {
edges.add(new ArrayList<>());
}
}
public void addEdge(int start, int end) { // 간선 추가
edges.get(start).add(end);
edges.get(end).add(start);
}
public void printGraph() { // 그래프 출력
for(int i=0;i<edges.size()-1;i++) {
System.out.print("Vertex "+i+"의 인접리스트 -> ");
for(int j=0;j<edges.get(i).size();j++) {
System.out.print(edges.get(i).get(j)+" ");
}
System.out.println();
}
}
public void clearVisitedArr() { // 방문 배열 초기화
Arrays.fill(visited, false);
}
public void bfs(int start) { // bfs 탐색
Queue<Integer> q = new LinkedList<>();
q.add(start);
visited[start] = true;
while(!q.isEmpty()) {
int cur = q.poll();
System.out.print(cur+" ");
for(int i=0;i<edges.get(cur).size();i++) {
int next = edges.get(cur).get(i);
if(!visited[next]) {
visited[next] = true;
q.add(next);
}
}
}
}
}
public class BfsSearch {
public static void main(String[] args) {
Graph graph = new Graph(7);
graph.addEdge(0, 1);
graph.addEdge(0, 2);
graph.addEdge(1, 3);
graph.addEdge(1, 4);
graph.addEdge(2, 4);
graph.addEdge(3, 6);
graph.addEdge(4, 6);
graph.addEdge(5, 6);
graph.printGraph();
graph.clearVisitedArr();
System.out.print("정점 0부터 탐색: ");
graph.bfs(0);
System.out.println();
}
}
'컴퓨터과학 > 자료구조&알고리즘' 카테고리의 다른 글
[자료구조와 알고리즘] 그래프 순회 - 깊이 우선 탐색 (dfs) (0) | 2021.12.29 |
---|---|
[자료구조와 알고리즘] 그래프 표현방법 (0) | 2021.12.16 |
[자료구조와 알고리즘] 그래프(Graph) 개념 (0) | 2021.12.16 |
Comments